Get Started with Arduino
This page will walk you through the basic moduals of FLEXIDOTS, and help you create your first wearable hardware experience with Arduino!
Components List
Microcontroller:
- Arduino Nano 33 BLE
- Arduino Nano Shield Module
Input / Output
- Button Module
-
Vibration Motor Module
Power
- 9V Battery Module
Attachment
Band*1
Connector*1
If you do not have all the above components, please refer to Hardware page to get the kit.
Circuit Basics
For the first example, you will build a basic hardware system including a Microcontroller, one Input, and one Output.
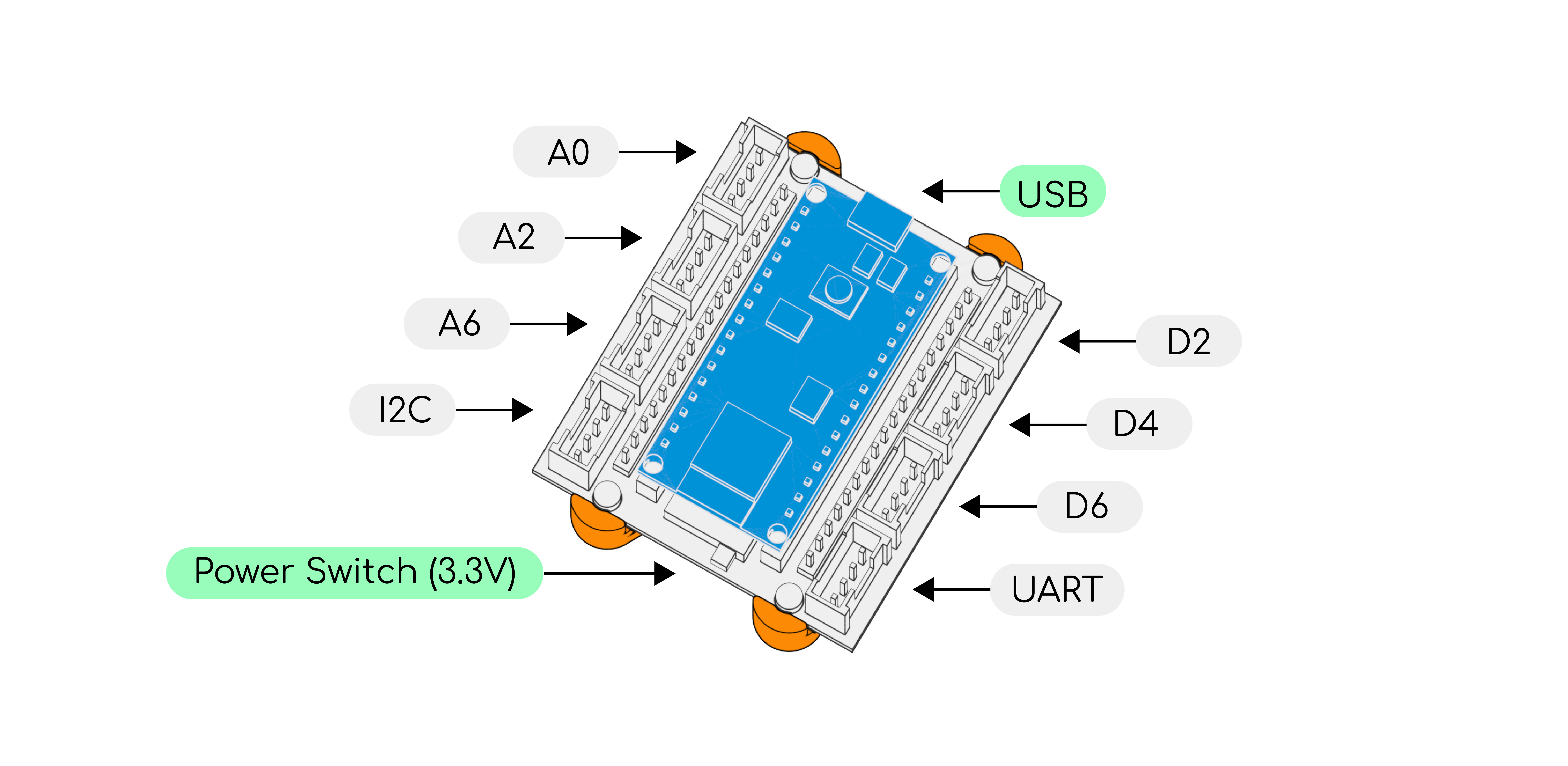
This example uses the Arduino Nano 33 BLE microcontroller. The shield provides extensions to different types of pins: D2/D4/D6 are digital pins that can input or output high and low (1/0) statuses. A0/A2/A6 are analog pins that can input or output analog values. And I2C and UART pins are special ports for certain protocols.
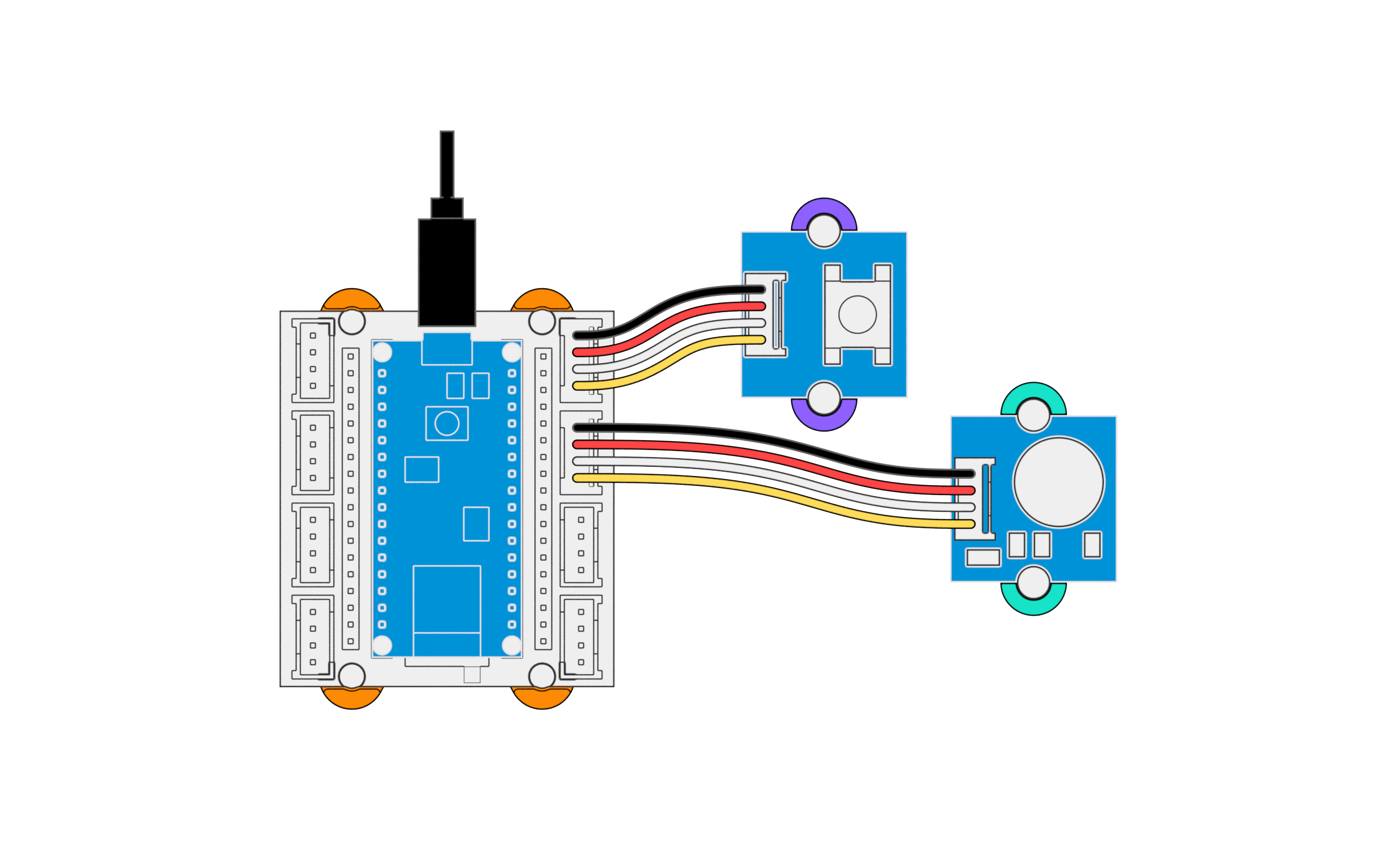
1️⃣ Connect the Button to D2, and the Vibration Motor to D4. We are also using the on-board LED, which does not require an external module to be connected.
Coding Basics
2️⃣ Now you can open the Arduino IDE to upload the code. Please make sure that the Arduino IDE has the Mbed OS core for Nano boards installed. Also note that this example is using the Arduino Nano 33 BLE board, so be sure to compile the code for the correct model.
To install and set up the Arduino IDE for the Arduino Nano 33 BLE, please refer to the official Arduino documentation.
3️⃣Connect the Arduino with your computer with USB cable, then select the correct port in the Tools Menu. Now you can upload the code, you can also download the code here.
const int buttonPin = 2; // set buttonPin to D2
const int ledPin = LED_BUILTIN; // set ledPin to on-board LED
const int vibrationMotorPin = 4; // set vibrationMotorPin to D4
// the setup function runs once when you press reset or power the board
void setup() {
pinMode(ledPin, OUTPUT); // use the LED as an output
pinMode(vibrationMotorPin, OUTPUT); // use the vibration motor as an output
pinMode(buttonPin, INPUT); // use button pin as an input
}
// the loop function runs over and over again forever
void loop() {
//detect the status from button
if (digitalRead(buttonPin) == HIGH) {
// turn LED/Vibration on:
digitalWrite(ledPin, HIGH);
digitalWrite(vibrationMotorPin, HIGH);
} else {
// turn LED/Vibration off:
digitalWrite(ledPin, LOW);
digitalWrite(vibrationMotorPin, LOW);
}
}
The Arduino code is separated into two basic parts: setup and loop. The setup function runs once when you press reset or power the board, and the loop function runs over and over again forever. Before the setup, we define several variables to help us handle the data in the code.
If you press the button, the on-board LED and the vibration motor will be activated.
Amazing Wearable
4️⃣ To begin with, use your imagination to assemble the circuit you just built on the FLEXIDOTS band! You can snap the buttons on the electronic components to the holes on the band.
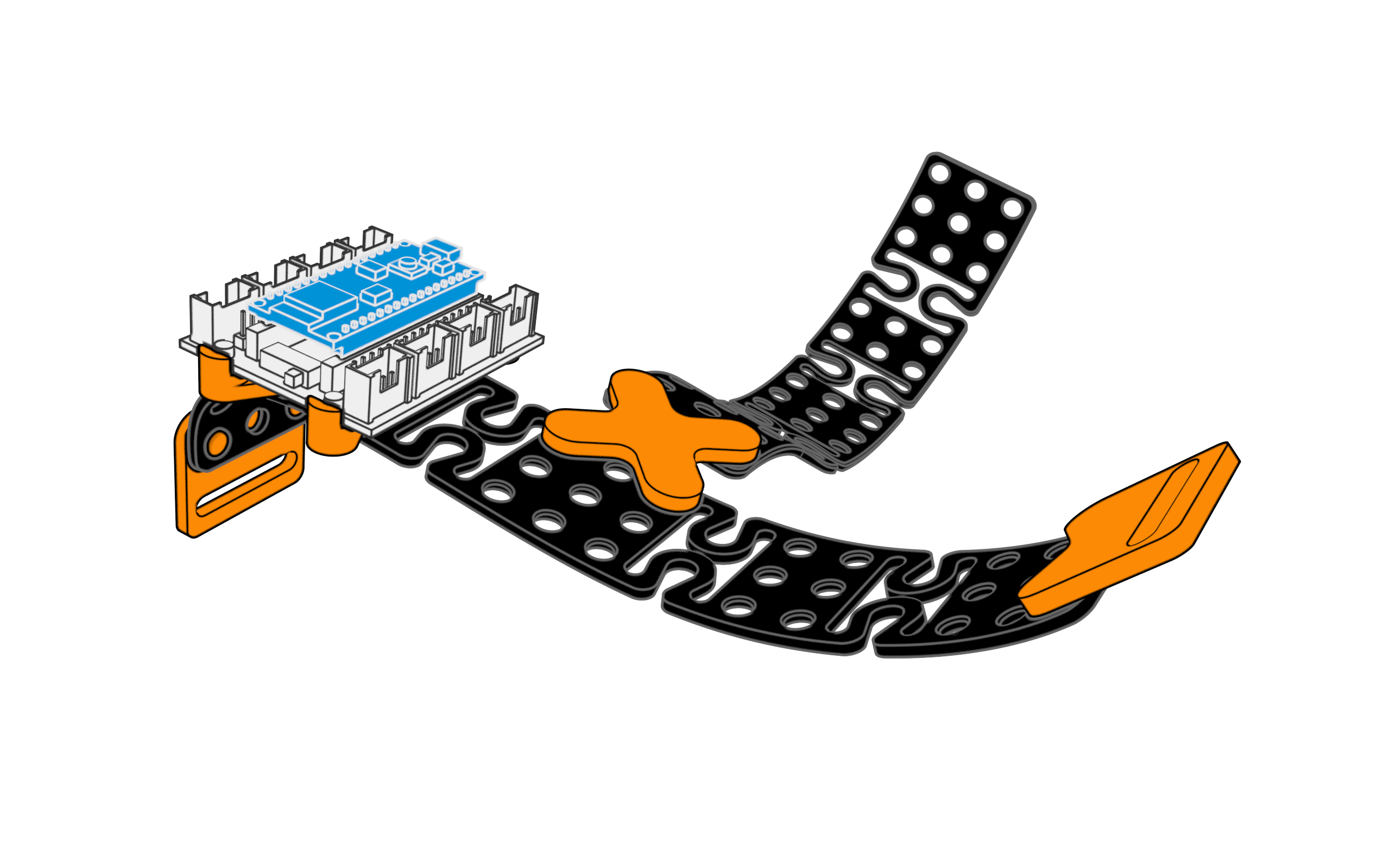
When designing a wearable device, we often want to incorporate data from our body, such as body temperature, heart rate, and body movement. The benefit of using the Arduino Nano 33 BLE is that it has an on-board IMU (gyroscopes + accelerometers + magnetometer), which allows us to easily detect body movement. For this example, we will use an additional library to read the data from the IMU. Please install the LSM9DS1 library from the library manager, you can refer to the official Arduino documentation.
5️⃣ In this example, we are reading accelerometer data from the IMU sensor. If the board tilts towards the X and Y axes, the vibration motor and LED will be triggered. Now you can open the Arduino IDE to upload the code, you can also download the code here.
//for accessing IMU sensor we need to load LSM9DS1 library
#include
float x, y, z; //set the variables for three axles
const int ledPin = LED_BUILTIN; // set ledPin to on-board LED
const int vibrationMotorPin = 4; // set vibrationMotorPin to D4
void setup() {
Serial.begin(9600);
if (!IMU.begin()) {
Serial.println("Failed to initialize IMU!");
while (1);
}
Serial.print("Accelerometer sample rate = ");
Serial.print(IMU.accelerationSampleRate());
Serial.println("Hz");
pinMode(ledPin, OUTPUT); // use the LED as an output
pinMode(vibrationMotorPin, OUTPUT); // use the vibration motor as an output
}
void loop() {
//read x y z value
if (IMU.accelerationAvailable()) {
IMU.readAcceleration(x, y, z);
}
//detect tilting
if (x > 0.1 || x < -0.1 || y > 0.1 ||y < -0.1) {
// turn LED/Vibration on:
digitalWrite(ledPin, HIGH);
digitalWrite(vibrationMotorPin, HIGH);
Serial.println("Tilting!");
}else{
// turn LED/Vibration off:
digitalWrite(ledPin, LOW);
digitalWrite(vibrationMotorPin, LOW);
}
delay(200);
}
6️⃣ Now you can unplug the FLEXIDOTS system from the computer, use the 9V Battery Module to power the board, and put the band on your wrist. Plug the red wire into the VIN pin and the black wire into the GND pin between the white sockets and the microcontroller. If you turn on the switch on the battery case, the entire hardware will run stand-alone.
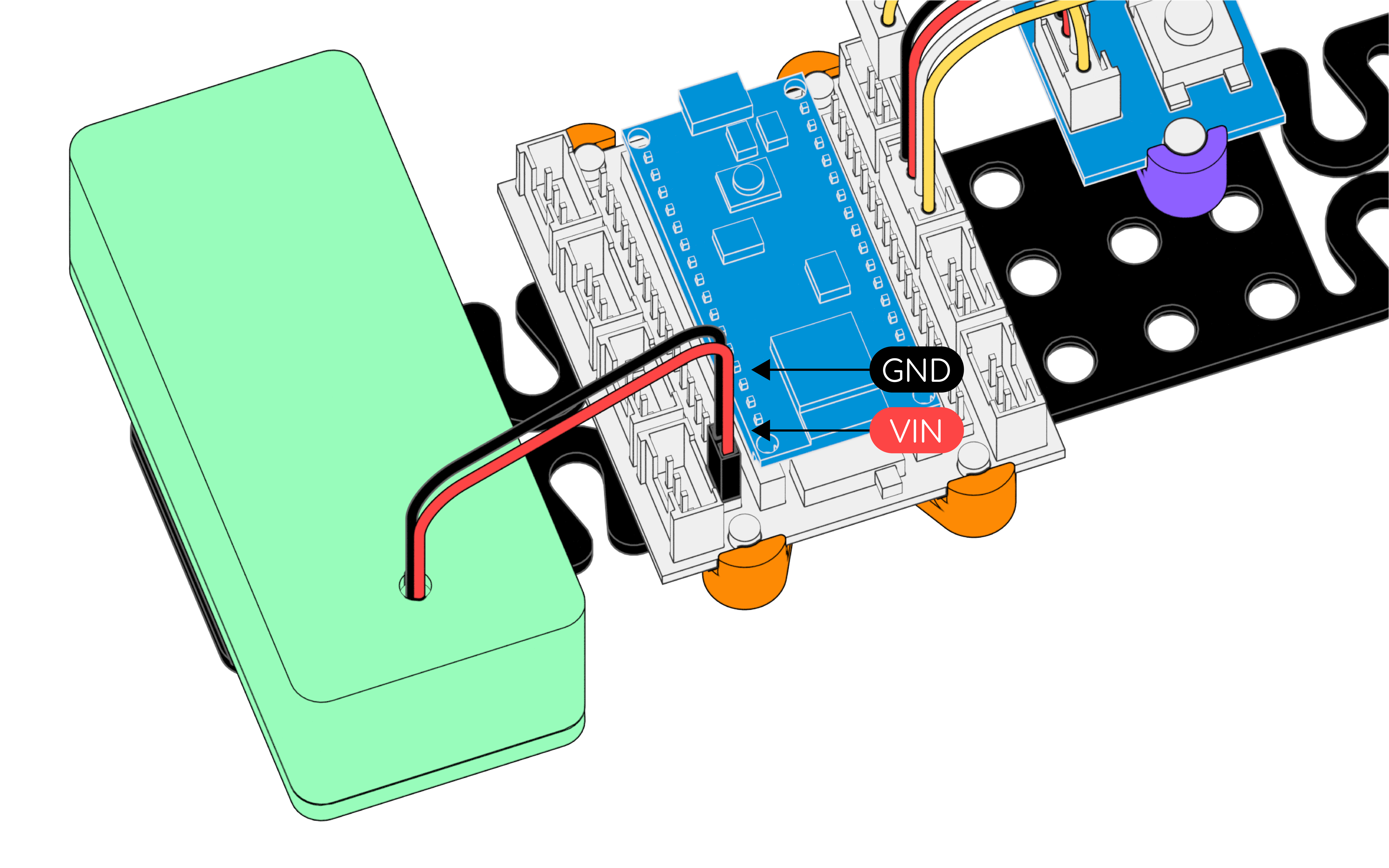
Let's see who has the steadiest hands!